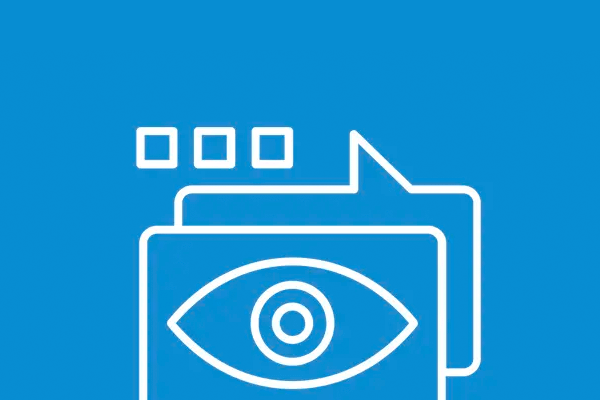
wp_update_plugins ( $extra_stats = array() )
wp_update_plugins是一个更新所有安装在WordPress网站上的插件的函数: 该函数检查WordPress插件库的更新,并在必要时应用它们: 该函数用于确保网站上安装的所有插件都是最新的,并具有最新的安全修复和功能。
根据WordPress.org上托管的最新版本,检查插件的可用更新。
尽管它的名字是这样,这个函数实际上并不执行任何更新,它只是检查可用的更新。
一个已安装的所有插件的列表将被发送到WP,同时还有网站的定位。
检查位于api.wordpress.org的WordPress服务器。只会在WordPress没有安装时进行检查。
function wp_update_plugins( $extra_stats = array() ) { if ( wp_installing() ) { return; } // Include an unmodified $wp_version. require ABSPATH . WPINC . '/version.php'; // If running blog-side, bail unless we've not checked in the last 12 hours. if ( ! function_exists( 'get_plugins' ) ) { require_once ABSPATH . 'wp-admin/includes/plugin.php'; } $plugins = get_plugins(); $translations = wp_get_installed_translations( 'plugins' ); $active = get_option( 'active_plugins', array() ); $current = get_site_transient( 'update_plugins' ); if ( ! is_object( $current ) ) { $current = new stdClass; } $updates = new stdClass; $updates->last_checked = time(); $updates->response = array(); $updates->translations = array(); $updates->no_update = array(); $doing_cron = wp_doing_cron(); // Check for update on a different schedule, depending on the page. switch ( current_filter() ) { case 'upgrader_process_complete': $timeout = 0; break; case 'load-update-core.php': $timeout = MINUTE_IN_SECONDS; break; case 'load-plugins.php': case 'load-update.php': $timeout = HOUR_IN_SECONDS; break; default: if ( $doing_cron ) { $timeout = 2 * HOUR_IN_SECONDS; } else { $timeout = 12 * HOUR_IN_SECONDS; } } $time_not_changed = isset( $current->last_checked ) && $timeout > ( time() - $current->last_checked ); if ( $time_not_changed && ! $extra_stats ) { $plugin_changed = false; foreach ( $plugins as $file => $p ) { $updates->checked[ $file ] = $p['Version']; if ( ! isset( $current->checked[ $file ] ) || (string) $current->checked[ $file ] !== (string) $p['Version'] ) { $plugin_changed = true; } } if ( isset( $current->response ) && is_array( $current->response ) ) { foreach ( $current->response as $plugin_file => $update_details ) { if ( ! isset( $plugins[ $plugin_file ] ) ) { $plugin_changed = true; break; } } } // Bail if we've checked recently and if nothing has changed. if ( ! $plugin_changed ) { return; } } // Update last_checked for current to prevent multiple blocking requests if request hangs. $current->last_checked = time(); set_site_transient( 'update_plugins', $current ); $to_send = compact( 'plugins', 'active' ); $locales = array_values( get_available_languages() ); /** * Filters the locales requested for plugin translations. * * @since 3.7.0 * @since 4.5.0 The default value of the `$locales` parameter changed to include all locales. * * @param string[] $locales Plugin locales. Default is all available locales of the site. */ $locales = apply_filters( 'plugins_update_check_locales', $locales ); $locales = array_unique( $locales ); if ( $doing_cron ) { $timeout = 30; // 30 seconds. } else { // Three seconds, plus one extra second for every 10 plugins. $timeout = 3 + (int) ( count( $plugins ) / 10 ); } $options = array( 'timeout' => $timeout, 'body' => array( 'plugins' => wp_json_encode( $to_send ), 'translations' => wp_json_encode( $translations ), 'locale' => wp_json_encode( $locales ), 'all' => wp_json_encode( true ), ), 'user-agent' => 'WordPress/' . $wp_version . '; ' . home_url( '/' ), ); if ( $extra_stats ) { $options['body']['update_stats'] = wp_json_encode( $extra_stats ); } $url = 'http://api.wordpress.org/plugins/update-check/1.1/'; $http_url = $url; $ssl = wp_http_supports( array( 'ssl' ) ); if ( $ssl ) { $url = set_url_scheme( $url, 'https' ); } $raw_response = wp_remote_post( $url, $options ); if ( $ssl && is_wp_error( $raw_response ) ) { trigger_error( sprintf( /* translators: %s: Support forums URL. */ __( 'An unexpected error occurred. Something may be wrong with WordPress.org or this server’s configuration. If you continue to have problems, please try the <a href="%s">support forums</a>.' ), __( 'https://wordpress.org/support/forums/' ) ) . ' ' . __( '(WordPress could not establish a secure connection to WordPress.org. Please contact your server administrator.)' ), headers_sent() || WP_DEBUG ? E_USER_WARNING : E_USER_NOTICE ); $raw_response = wp_remote_post( $http_url, $options ); } if ( is_wp_error( $raw_response ) || 200 !== wp_remote_retrieve_response_code( $raw_response ) ) { return; } $response = json_decode( wp_remote_retrieve_body( $raw_response ), true ); if ( $response && is_array( $response ) ) { $updates->response = $response['plugins']; $updates->translations = $response['translations']; $updates->no_update = $response['no_update']; } // Support updates for any plugins using the `Update URI` header field. foreach ( $plugins as $plugin_file => $plugin_data ) { if ( ! $plugin_data['UpdateURI'] || isset( $updates->response[ $plugin_file ] ) ) { continue; } $hostname = wp_parse_url( sanitize_url( $plugin_data['UpdateURI'] ), PHP_URL_HOST ); /** * Filters the update response for a given plugin hostname. * * The dynamic portion of the hook name, `$hostname`, refers to the hostname * of the URI specified in the `Update URI` header field. * * @since 5.8.0 * * @param array|false $update { * The plugin update data with the latest details. Default false. * * @type string $id Optional. ID of the plugin for update purposes, should be a URI * specified in the `Update URI` header field. * @type string $slug Slug of the plugin. * @type string $version The version of the plugin. * @type string $url The URL for details of the plugin. * @type string $package Optional. The update ZIP for the plugin. * @type string $tested Optional. The version of WordPress the plugin is tested against. * @type string $requires_php Optional. The version of PHP which the plugin requires. * @type bool $autoupdate Optional. Whether the plugin should automatically update. * @type array $icons Optional. Array of plugin icons. * @type array $banners Optional. Array of plugin banners. * @type array $banners_rtl Optional. Array of plugin RTL banners. * @type array $translations { * Optional. List of translation updates for the plugin. * * @type string $language The language the translation update is for. * @type string $version The version of the plugin this translation is for. * This is not the version of the language file. * @type string $updated The update timestamp of the translation file. * Should be a date in the `YYYY-MM-DD HH:MM:SS` format. * @type string $package The ZIP location containing the translation update. * @type string $autoupdate Whether the translation should be automatically installed. * } * } * @param array $plugin_data Plugin headers. * @param string $plugin_file Plugin filename. * @param string[] $locales Installed locales to look up translations for. */ $update = apply_filters( "update_plugins_{$hostname}", false, $plugin_data, $plugin_file, $locales ); if ( ! $update ) { continue; } $update = (object) $update; // Is it valid? We require at least a version. if ( ! isset( $update->version ) ) { continue; } // These should remain constant. $update->id = $plugin_data['UpdateURI']; $update->plugin = $plugin_file; // WordPress needs the version field specified as 'new_version'. if ( ! isset( $update->new_version ) ) { $update->new_version = $update->version; } // Handle any translation updates. if ( ! empty( $update->translations ) ) { foreach ( $update->translations as $translation ) { if ( isset( $translation['language'], $translation['package'] ) ) { $translation['type'] = 'plugin'; $translation['slug'] = isset( $update->slug ) ? $update->slug : $update->id; $updates->translations[] = $translation; } } } unset( $updates->no_update[ $plugin_file ], $updates->response[ $plugin_file ] ); if ( version_compare( $update->new_version, $plugin_data['Version'], '>' ) ) { $updates->response[ $plugin_file ] = $update; } else { $updates->no_update[ $plugin_file ] = $update; } } $sanitize_plugin_update_payload = static function( &$item ) { $item = (object) $item; unset( $item->translations, $item->compatibility ); return $item; }; array_walk( $updates->response, $sanitize_plugin_update_payload ); array_walk( $updates->no_update, $sanitize_plugin_update_payload ); set_site_transient( 'update_plugins', $updates ); }
要使用` get_users
`函数获取所有用户列表,可以按照以下步骤进行:
1. 使用` get_users
`函数调用获取用户列表:
$users = get_users();
2. 您可以按需使用参数来过滤结果。例如,您可以通过角色、用户ID、用户登录名等过滤用户列表。以下是一个根据用户角色为过滤条件的示例:
$users = get_users( array( 'role' => 'subscriber' // 将角色名称替换为您要过滤的角色 ) );
在上述示例中,将` role
`参数设置为所需的角色名称来过滤用户列表。
3. 您可以使用循环遍历获取的用户列表,并访问每个用户的属性。例如,以下示例将显示每个用户的用户名和电子邮件地址:
foreach( $users as $user ) { echo '用户名:' . $user->user_login . ', 电子邮件:' . $user->user_email . ; }
在上述示例中,通过` $user->user_login
`和` $user->user_email
`访问每个用户的用户名和电子邮件地址。
请注意,` get_users
`函数默认返回所有用户,并可以根据需要使用更多参数进行过滤。您可以参阅WordPress官方文档中的` get_users
`函数文档,了解更多可用参数和用法示例。
总结起来,使用` get_users
`函数获取所有用户列表的步骤是:
get_users
`函数获取用户列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量来定义插件的目录路径和URL。
1. `WP_PLUGIN_DIR`:这是一个常量,用于定义插件的目录路径(文件系统路径)。您可以使用以下代码在插件文件中访问该常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述代码中,将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件文件的完整路径。
2. `WP_PLUGIN_URL`:这是一个常量,用于定义插件的URL(用于在网页上访问插件文件)。以下是一个使用该常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同样,请将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件在网页上的完整URL。
请注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之后引入。从WordPress 5.5版本开始,这两个常量被标记为过时(deprecated),因为WordPress更倾向于使用新的插件文件结构。如果您正在开发新插件,建议使用新的插件文件结构和相关函数。
在新的插件文件结构中,可以使用以下函数来获取插件的目录路径和URL:
- `plugin_dir_path()`:获取插件目录路径。
- `plugin_dir_url()`:获取插件URL。
这些函数会自动将插件的版本、多站点和SSL等考虑因素纳入计算。
总结起来,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定义插件的目录和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但请注意,这两个常量已被标记为过时,建议使用新的插件文件结构和相关函数来获取插件的路径和URL。
使用PHP在WordPress中添加自定义功能可以通过以下方式实现:
下面是一个实操示例。
要在WordPress中添加自定义功能,可以按照以下步骤使用PHP编写并添加自定义功能:
// 添加自定义功能示例 // 1. 创建自定义短代码 function custom_shortcode() { return '这是我的自定义短代码内容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定义小工具 function custom_widget() { echo '这是我的自定义小工具内容'; } register_widget('custom_widget'); // 3. 自定义菜单 function custom_menu() { register_nav_menu('custom-menu', '自定义菜单'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定义页面模板 function custom_page_template() { /* Template Name: 自定义模板 */ // 自定义模板的内容和样式 }
请注意,修改主题文件可以在主题更新时丢失,因此建议在进行任何更改之前备份functions.php文件。此外,为避免不必要的错误和冲突,建议在添加自定义功能前先了解WordPress开发文档和最佳实践,以确保正确、安全地实现所需的自定义功能。
使用 do_action
函数可以触发一个钩子函数。do_action
函数的参数与要触发的钩子函数的参数相同。
例如,触发save_post钩子函数的代码如下:
do_action( 'save_post', $post_ID, $post );
这里,$post_ID
和 $post
是传递给钩子函数的参数。
使用 wp_get_current_user
获取当前登录用户的信息:
$current_user = wp_get_current_user(); // 获取当前用户的ID $user_id = $current_user->ID; // 获取当前用户的用户名 $user_login = $current_user->user_login; // 获取当前用户的邮箱 $user_email = $current_user->user_email; // 获取当前用户的显示名称 $display_name = $current_user->display_name;