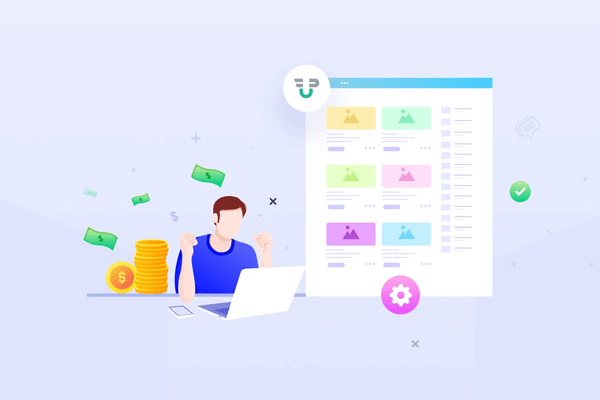
wpmu_validate_blog_signup ( $blogname, $blog_title, $user = '' )
wpmu_validate_blog_signup: 这个函数在WPMU安装中用来验证一个新博客的注册细节。它以博客域名、博客路径和标题为参数,并返回一个数组,其中包含验证过程中发生的任何错误的细节: 这个函数可以被过滤,以允许在博客注册过程中添加自定义的验证规则。
处理新网站的注册。
检查用户在博客注册时提供的数据。验证博客路径和域名的有效性和唯一性。
这个函数可以防止当前用户用相当于另一个用户的登录名的模糊名字来注册一个新的网站。向该函数传递$user参数,其中$user是其他用户,实际上是对这一限制的覆盖。
过滤 {@see ‘wpmu_validate_blog_signup’} 如果你想修改WordPress验证新网站注册的方式。
function wpmu_validate_blog_signup( $blogname, $blog_title, $user = '' ) { global $wpdb, $domain; $current_network = get_network(); $base = $current_network->path; $blog_title = strip_tags( $blog_title ); $errors = new WP_Error(); $illegal_names = get_site_option( 'illegal_names' ); if ( false == $illegal_names ) { $illegal_names = array( 'www', 'web', 'root', 'admin', 'main', 'invite', 'administrator' ); add_site_option( 'illegal_names', $illegal_names ); } /* * On sub dir installations, some names are so illegal, only a filter can * spring them from jail. */ if ( ! is_subdomain_install() ) { $illegal_names = array_merge( $illegal_names, get_subdirectory_reserved_names() ); } if ( empty( $blogname ) ) { $errors->add( 'blogname', __( 'Please enter a site name.' ) ); } if ( preg_match( '/[^a-z0-9]+/', $blogname ) ) { $errors->add( 'blogname', __( 'Site names can only contain lowercase letters (a-z) and numbers.' ) ); } if ( in_array( $blogname, $illegal_names, true ) ) { $errors->add( 'blogname', __( 'That name is not allowed.' ) ); } /** * Filters the minimum site name length required when validating a site signup. * * @since 4.8.0 * * @param int $length The minimum site name length. Default 4. */ $minimum_site_name_length = apply_filters( 'minimum_site_name_length', 4 ); if ( strlen( $blogname ) < $minimum_site_name_length ) { /* translators: %s: Minimum site name length. */ $errors->add( 'blogname', sprintf( _n( 'Site name must be at least %s character.', 'Site name must be at least %s characters.', $minimum_site_name_length ), number_format_i18n( $minimum_site_name_length ) ) ); } // Do not allow users to create a site that conflicts with a page on the main blog. if ( ! is_subdomain_install() && $wpdb->get_var( $wpdb->prepare( 'SELECT post_name FROM ' . $wpdb->get_blog_prefix( $current_network->site_id ) . "posts WHERE post_type = 'page' AND post_name = %s", $blogname ) ) ) { $errors->add( 'blogname', __( 'Sorry, you may not use that site name.' ) ); } // All numeric? if ( preg_match( '/^[0-9]*$/', $blogname ) ) { $errors->add( 'blogname', __( 'Sorry, site names must have letters too!' ) ); } /** * Filters the new site name during registration. * * The name is the site's subdomain or the site's subdirectory * path depending on the network settings. * * @since MU (3.0.0) * * @param string $blogname Site name. */ $blogname = apply_filters( 'newblogname', $blogname ); $blog_title = wp_unslash( $blog_title ); if ( empty( $blog_title ) ) { $errors->add( 'blog_title', __( 'Please enter a site title.' ) ); } // Check if the domain/path has been used already. if ( is_subdomain_install() ) { $mydomain = $blogname . '.' . preg_replace( '|^www.|', '', $domain ); $path = $base; } else { $mydomain = $domain; $path = $base . $blogname . '/'; } if ( domain_exists( $mydomain, $path, $current_network->id ) ) { $errors->add( 'blogname', __( 'Sorry, that site already exists!' ) ); } /* * Do not allow users to create a site that matches an existing user's login name, * unless it's the user's own username. */ if ( username_exists( $blogname ) ) { if ( ! is_object( $user ) || ( is_object( $user ) && ( $user->user_login != $blogname ) ) ) { $errors->add( 'blogname', __( 'Sorry, that site is reserved!' ) ); } } // Has someone already signed up for this domain? // TODO: Check email too? $signup = $wpdb->get_row( $wpdb->prepare( "SELECT * FROM $wpdb->signups WHERE domain = %s AND path = %s", $mydomain, $path ) ); if ( $signup instanceof stdClass ) { $diff = time() - mysql2date( 'U', $signup->registered ); // If registered more than two days ago, cancel registration and let this signup go through. if ( $diff > 2 * DAY_IN_SECONDS ) { $wpdb->delete( $wpdb->signups, array( 'domain' => $mydomain, 'path' => $path, ) ); } else { $errors->add( 'blogname', __( 'That site is currently reserved but may be available in a couple days.' ) ); } } $result = array( 'domain' => $mydomain, 'path' => $path, 'blogname' => $blogname, 'blog_title' => $blog_title, 'user' => $user, 'errors' => $errors, ); /** * Filters site details and error messages following registration. * * @since MU (3.0.0) * * @param array $result { * Array of domain, path, blog name, blog title, user and error messages. * * @type string $domain Domain for the site. * @type string $path Path for the site. Used in subdirectory installations. * @type string $blogname The unique site name (slug). * @type string $blog_title Blog title. * @type string|WP_User $user By default, an empty string. A user object if provided. * @type WP_Error $errors WP_Error containing any errors found. * } */ return apply_filters( 'wpmu_validate_blog_signup', $result ); }
要使用` get_users
`函数获取所有用户列表,可以按照以下步骤进行:
1. 使用` get_users
`函数调用获取用户列表:
$users = get_users();
2. 您可以按需使用参数来过滤结果。例如,您可以通过角色、用户ID、用户登录名等过滤用户列表。以下是一个根据用户角色为过滤条件的示例:
$users = get_users( array( 'role' => 'subscriber' // 将角色名称替换为您要过滤的角色 ) );
在上述示例中,将` role
`参数设置为所需的角色名称来过滤用户列表。
3. 您可以使用循环遍历获取的用户列表,并访问每个用户的属性。例如,以下示例将显示每个用户的用户名和电子邮件地址:
foreach( $users as $user ) { echo '用户名:' . $user->user_login . ', 电子邮件:' . $user->user_email . ; }
在上述示例中,通过` $user->user_login
`和` $user->user_email
`访问每个用户的用户名和电子邮件地址。
请注意,` get_users
`函数默认返回所有用户,并可以根据需要使用更多参数进行过滤。您可以参阅WordPress官方文档中的` get_users
`函数文档,了解更多可用参数和用法示例。
总结起来,使用` get_users
`函数获取所有用户列表的步骤是:
get_users
`函数获取用户列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量来定义插件的目录路径和URL。
1. `WP_PLUGIN_DIR`:这是一个常量,用于定义插件的目录路径(文件系统路径)。您可以使用以下代码在插件文件中访问该常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述代码中,将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件文件的完整路径。
2. `WP_PLUGIN_URL`:这是一个常量,用于定义插件的URL(用于在网页上访问插件文件)。以下是一个使用该常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同样,请将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件在网页上的完整URL。
请注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之后引入。从WordPress 5.5版本开始,这两个常量被标记为过时(deprecated),因为WordPress更倾向于使用新的插件文件结构。如果您正在开发新插件,建议使用新的插件文件结构和相关函数。
在新的插件文件结构中,可以使用以下函数来获取插件的目录路径和URL:
- `plugin_dir_path()`:获取插件目录路径。
- `plugin_dir_url()`:获取插件URL。
这些函数会自动将插件的版本、多站点和SSL等考虑因素纳入计算。
总结起来,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定义插件的目录和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但请注意,这两个常量已被标记为过时,建议使用新的插件文件结构和相关函数来获取插件的路径和URL。
使用PHP在WordPress中添加自定义功能可以通过以下方式实现:
下面是一个实操示例。
要在WordPress中添加自定义功能,可以按照以下步骤使用PHP编写并添加自定义功能:
// 添加自定义功能示例 // 1. 创建自定义短代码 function custom_shortcode() { return '这是我的自定义短代码内容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定义小工具 function custom_widget() { echo '这是我的自定义小工具内容'; } register_widget('custom_widget'); // 3. 自定义菜单 function custom_menu() { register_nav_menu('custom-menu', '自定义菜单'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定义页面模板 function custom_page_template() { /* Template Name: 自定义模板 */ // 自定义模板的内容和样式 }
请注意,修改主题文件可以在主题更新时丢失,因此建议在进行任何更改之前备份functions.php文件。此外,为避免不必要的错误和冲突,建议在添加自定义功能前先了解WordPress开发文档和最佳实践,以确保正确、安全地实现所需的自定义功能。
使用 do_action
函数可以触发一个钩子函数。do_action
函数的参数与要触发的钩子函数的参数相同。
例如,触发save_post钩子函数的代码如下:
do_action( 'save_post', $post_ID, $post );
这里,$post_ID
和 $post
是传递给钩子函数的参数。
使用 wp_get_current_user
获取当前登录用户的信息:
$current_user = wp_get_current_user(); // 获取当前用户的ID $user_id = $current_user->ID; // 获取当前用户的用户名 $user_login = $current_user->user_login; // 获取当前用户的邮箱 $user_email = $current_user->user_email; // 获取当前用户的显示名称 $display_name = $current_user->display_name;