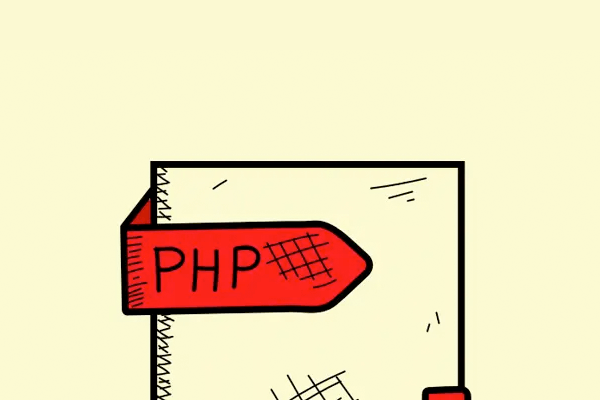
wp_get_loading_optimization_attributes ( $tag_name, $attr, $context )
获取加载优化属性。此函数返回一个属性数组,这些属性应合并到给定的属性数组中,以优化加载性能。此函数返回的潜在属性包括:
– 值为 “lazy” 的 `loading` 属性
– 取值为 “high” 的 `fetchpriority` 属性
– 值为 “async” 的 `decoding` 属性
如果这些属性中的任何一个已经存在于给定的属性中,它们将不会被修改。请注意,任何元素都不应同时具有 `loading=”lazy”` 和 `fetchpriority=”high”` 属性,因此,如果这两个属性的值都存在,函数将触发警告。
function wp_get_loading_optimization_attributes( $tag_name, $attr, $context ) { global $wp_query; /** * Filters whether to short-circuit loading optimization attributes. * * Returning an array from the filter will effectively short-circuit the loading of optimization attributes, * returning that value instead. * * @since 6.4.0 * * @param array|false $loading_attrs False by default, or array of loading optimization attributes to short-circuit. * @param string $tag_name The tag name. * @param array $attr Array of the attributes for the tag. * @param string $context Context for the element for which the loading optimization attribute is requested. */ $loading_attrs = apply_filters( 'pre_wp_get_loading_optimization_attributes', false, $tag_name, $attr, $context ); if ( is_array( $loading_attrs ) ) { return $loading_attrs; } $loading_attrs = array(); /* * Skip lazy-loading for the overall block template, as it is handled more granularly. * The skip is also applicable for `fetchpriority`. */ if ( 'template' === $context ) { /** This filter is documented in wp-includes/media.php */ return apply_filters( 'wp_get_loading_optimization_attributes', $loading_attrs, $tag_name, $attr, $context ); } // For now this function only supports images and iframes. if ( 'img' !== $tag_name && 'iframe' !== $tag_name ) { /** This filter is documented in wp-includes/media.php */ return apply_filters( 'wp_get_loading_optimization_attributes', $loading_attrs, $tag_name, $attr, $context ); } /* * Skip programmatically created images within content blobs as they need to be handled together with the other * images within the post content or widget content. * Without this clause, they would already be considered within their own context which skews the image count and * can result in the first post content image being lazy-loaded or an image further down the page being marked as a * high priority. */ if ( 'the_content' !== $context && doing_filter( 'the_content' ) || 'widget_text_content' !== $context && doing_filter( 'widget_text_content' ) || 'widget_block_content' !== $context && doing_filter( 'widget_block_content' ) ) { /** This filter is documented in wp-includes/media.php */ return apply_filters( 'wp_get_loading_optimization_attributes', $loading_attrs, $tag_name, $attr, $context ); } /* * Add `decoding` with a value of "async" for every image unless it has a * conflicting `decoding` attribute already present. */ if ( 'img' === $tag_name ) { if ( isset( $attr['decoding'] ) ) { $loading_attrs['decoding'] = $attr['decoding']; } else { $loading_attrs['decoding'] = 'async'; } } // For any resources, width and height must be provided, to avoid layout shifts. if ( ! isset( $attr['width'], $attr['height'] ) ) { /** This filter is documented in wp-includes/media.php */ return apply_filters( 'wp_get_loading_optimization_attributes', $loading_attrs, $tag_name, $attr, $context ); } /* * The key function logic starts here. */ $maybe_in_viewport = null; $increase_count = false; $maybe_increase_count = false; // Logic to handle a `loading` attribute that is already provided. if ( isset( $attr['loading'] ) ) { /* * Interpret "lazy" as not in viewport. Any other value can be * interpreted as in viewport (realistically only "eager" or `false` * to force-omit the attribute are other potential values). */ if ( 'lazy' === $attr['loading'] ) { $maybe_in_viewport = false; } else { $maybe_in_viewport = true; } } // Logic to handle a `fetchpriority` attribute that is already provided. if ( isset( $attr['fetchpriority'] ) && 'high' === $attr['fetchpriority'] ) { /* * If the image was already determined to not be in the viewport (e.g. * from an already provided `loading` attribute), trigger a warning. * Otherwise, the value can be interpreted as in viewport, since only * the most important in-viewport image should have `fetchpriority` set * to "high". */ if ( false === $maybe_in_viewport ) { _doing_it_wrong( __FUNCTION__, __( 'An image should not be lazy-loaded and marked as high priority at the same time.' ), '6.3.0' ); /* * Set `fetchpriority` here for backward-compatibility as we should * not override what a developer decided, even though it seems * incorrect. */ $loading_attrs['fetchpriority'] = 'high'; } else { $maybe_in_viewport = true; } } if ( null === $maybe_in_viewport ) { $header_enforced_contexts = array( 'template_part_' . WP_TEMPLATE_PART_AREA_HEADER => true, 'get_header_image_tag' => true, ); /** * Filters the header-specific contexts. * * @since 6.4.0 * * @param array $default_header_enforced_contexts Map of contexts for which elements should be considered * in the header of the page, as $context => $enabled * pairs. The $enabled should always be true. */ $header_enforced_contexts = apply_filters( 'wp_loading_optimization_force_header_contexts', $header_enforced_contexts ); // Consider elements with these header-specific contexts to be in viewport. if ( isset( $header_enforced_contexts[ $context ] ) ) { $maybe_in_viewport = true; $maybe_increase_count = true; } elseif ( ! is_admin() && in_the_loop() && is_main_query() ) { /* * Get the content media count, since this is a main query * content element. This is accomplished by "increasing" * the count by zero, as the only way to get the count is * to call this function. * The actual count increase happens further below, based * on the `$increase_count` flag set here. */ $content_media_count = wp_increase_content_media_count( 0 ); $increase_count = true; // If the count so far is below the threshold, `loading` attribute is omitted. if ( $content_media_count < wp_omit_loading_attr_threshold() ) { $maybe_in_viewport = true; } else { $maybe_in_viewport = false; } } elseif ( // Only apply for main query but before the loop. $wp_query->before_loop && $wp_query->is_main_query() /* * Any image before the loop, but after the header has started should not be lazy-loaded, * except when the footer has already started which can happen when the current template * does not include any loop. */ && did_action( 'get_header' ) && ! did_action( 'get_footer' ) ) { $maybe_in_viewport = true; $maybe_increase_count = true; } } /* * If the element is in the viewport (`true`), potentially add * `fetchpriority` with a value of "high". Otherwise, i.e. if the element * is not not in the viewport (`false`) or it is unknown (`null`), add * `loading` with a value of "lazy". */ if ( $maybe_in_viewport ) { $loading_attrs = wp_maybe_add_fetchpriority_high_attr( $loading_attrs, $tag_name, $attr ); } else { // Only add `loading="lazy"` if the feature is enabled. if ( wp_lazy_loading_enabled( $tag_name, $context ) ) { $loading_attrs['loading'] = 'lazy'; } } /* * If flag was set based on contextual logic above, increase the content * media count, either unconditionally, or based on whether the image size * is larger than the threshold. */ if ( $increase_count ) { wp_increase_content_media_count(); } elseif ( $maybe_increase_count ) { /** This filter is documented in wp-includes/media.php */ $wp_min_priority_img_pixels = apply_filters( 'wp_min_priority_img_pixels', 50000 ); if ( $wp_min_priority_img_pixels <= $attr['width'] * $attr['height'] ) { wp_increase_content_media_count(); } } /** * Filters the loading optimization attributes. * * @since 6.4.0 * * @param array $loading_attrs The loading optimization attributes. * @param string $tag_name The tag name. * @param array $attr Array of the attributes for the tag. * @param string $context Context for the element for which the loading optimization attribute is requested. */ return apply_filters( 'wp_get_loading_optimization_attributes', $loading_attrs, $tag_name, $attr, $context ); }
要使用` get_users
`函数获取所有用户列表,可以按照以下步骤进行:
1. 使用` get_users
`函数调用获取用户列表:
$users = get_users();
2. 您可以按需使用参数来过滤结果。例如,您可以通过角色、用户ID、用户登录名等过滤用户列表。以下是一个根据用户角色为过滤条件的示例:
$users = get_users( array( 'role' => 'subscriber' // 将角色名称替换为您要过滤的角色 ) );
在上述示例中,将` role
`参数设置为所需的角色名称来过滤用户列表。
3. 您可以使用循环遍历获取的用户列表,并访问每个用户的属性。例如,以下示例将显示每个用户的用户名和电子邮件地址:
foreach( $users as $user ) { echo '用户名:' . $user->user_login . ', 电子邮件:' . $user->user_email . ; }
在上述示例中,通过` $user->user_login
`和` $user->user_email
`访问每个用户的用户名和电子邮件地址。
请注意,` get_users
`函数默认返回所有用户,并可以根据需要使用更多参数进行过滤。您可以参阅WordPress官方文档中的` get_users
`函数文档,了解更多可用参数和用法示例。
总结起来,使用` get_users
`函数获取所有用户列表的步骤是:
get_users
`函数获取用户列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量来定义插件的目录路径和URL。
1. `WP_PLUGIN_DIR`:这是一个常量,用于定义插件的目录路径(文件系统路径)。您可以使用以下代码在插件文件中访问该常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述代码中,将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件文件的完整路径。
2. `WP_PLUGIN_URL`:这是一个常量,用于定义插件的URL(用于在网页上访问插件文件)。以下是一个使用该常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同样,请将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件在网页上的完整URL。
请注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之后引入。从WordPress 5.5版本开始,这两个常量被标记为过时(deprecated),因为WordPress更倾向于使用新的插件文件结构。如果您正在开发新插件,建议使用新的插件文件结构和相关函数。
在新的插件文件结构中,可以使用以下函数来获取插件的目录路径和URL:
- `plugin_dir_path()`:获取插件目录路径。
- `plugin_dir_url()`:获取插件URL。
这些函数会自动将插件的版本、多站点和SSL等考虑因素纳入计算。
总结起来,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定义插件的目录和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但请注意,这两个常量已被标记为过时,建议使用新的插件文件结构和相关函数来获取插件的路径和URL。
使用PHP在WordPress中添加自定义功能可以通过以下方式实现:
下面是一个实操示例。
要在WordPress中添加自定义功能,可以按照以下步骤使用PHP编写并添加自定义功能:
// 添加自定义功能示例 // 1. 创建自定义短代码 function custom_shortcode() { return '这是我的自定义短代码内容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定义小工具 function custom_widget() { echo '这是我的自定义小工具内容'; } register_widget('custom_widget'); // 3. 自定义菜单 function custom_menu() { register_nav_menu('custom-menu', '自定义菜单'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定义页面模板 function custom_page_template() { /* Template Name: 自定义模板 */ // 自定义模板的内容和样式 }
请注意,修改主题文件可以在主题更新时丢失,因此建议在进行任何更改之前备份functions.php文件。此外,为避免不必要的错误和冲突,建议在添加自定义功能前先了解WordPress开发文档和最佳实践,以确保正确、安全地实现所需的自定义功能。
使用 do_action
函数可以触发一个钩子函数。do_action
函数的参数与要触发的钩子函数的参数相同。
例如,触发save_post钩子函数的代码如下:
do_action( 'save_post', $post_ID, $post );
这里,$post_ID
和 $post
是传递给钩子函数的参数。
使用 wp_get_current_user
获取当前登录用户的信息:
$current_user = wp_get_current_user(); // 获取当前用户的ID $user_id = $current_user->ID; // 获取当前用户的用户名 $user_login = $current_user->user_login; // 获取当前用户的邮箱 $user_email = $current_user->user_email; // 获取当前用户的显示名称 $display_name = $current_user->display_name;